NumPy: numpy.dstack() function
numpy.dstack() function
The numpy.dstack() is used to stack arrays in sequence depth wise (along third axis).
This is equivalent to concatenation along the third axis after 2-D arrays of shape (M,N) have been reshaped to (M,N,1) and 1-D arrays of shape (N,) have been reshaped to (1,N,1). Rebuilds arrays divided by dsplit.
This function is particularly useful for working with image data, where each image is represented as a 2D array of pixel values, and a collection of images can be stacked depth-wise to create a 3D array of shape (height, width, number of images).
Syntax:
numpy.dstack(tup)
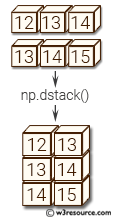
Parameter:
Name | Description | Required / Optional |
---|---|---|
tup | The arrays must have the same shape along all but the third axis. 1-D or 2-D arrays must have the same shape. | Required |
Return value:
stacked : ndarray The array formed by stacking the given arrays, will be at least 3-D.
Example: Concatenating Arrays Along the Third Axis with numpy.dstack()
>>> import numpy as np
>>> x = np.array((3, 5, 7))
>>> y = np.array((5, 7, 9))
>>> np.dstack((x,y))
array([[[3, 5],
[5, 7],
[7, 9]]])
In the above code, two 1-D arrays, x and y, are created using np.array() function. Then, np.dstack() function is used to stack these arrays horizontally along the third axis to create a 3-D array. The result is an array with shape (1, 3, 2), where the first dimension represents the number of arrays (1), the second dimension represents the length of the original arrays (3), and the third dimension represents the number of elements in each array (2).
Pictorial Presentation:
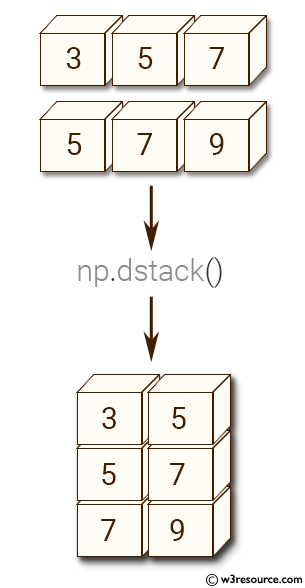
Example: Stacking 2D arrays using numpy.dstack()
>>> import numpy as np
>>> x = np.array([[3], [5], [7]])
>>> y = np.array([[5], [7], [9]])
>>> np.dstack((x,y))
array([[[3, 5]],
[[5, 7]],
[[7, 9]]])
In the above code two 2D arrays, 'x' and 'y', are created using numpy.array() function. These arrays have a shape of (3,1) which means they have 3 rows and 1 column.
The np.dstack() function is then used to stack the two arrays along the third dimension. This results in a new 3D array with a shape of (3,1,2). The first dimension represents the rows, the second dimension represents the columns, and the third dimension represents the stacked arrays.
Pictorial Presentation:
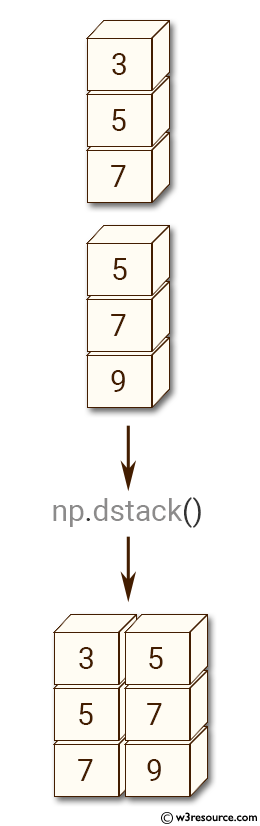
Python - NumPy Code Editor:
Previous: column_stack()
Next: hstack()
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics